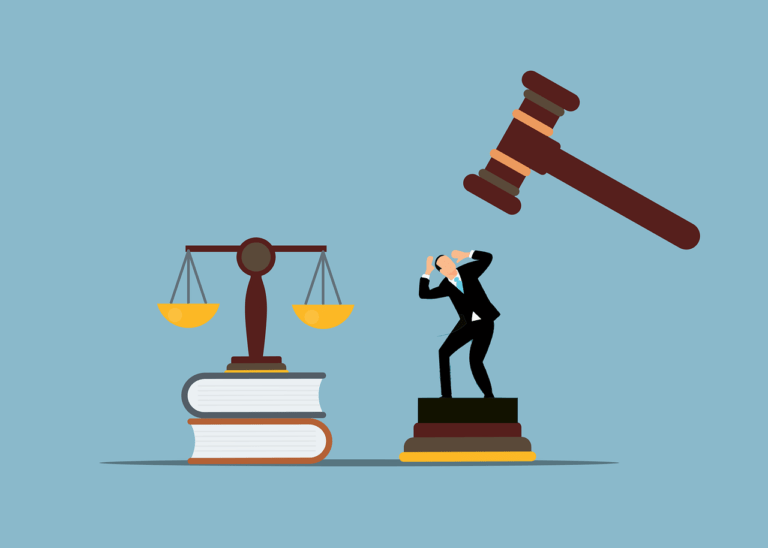
Three new laws have been enacted in India from July 1, 2024
Three new laws have been enacted in India, set to replace the colonial-era Indian Penal Code (IPC), Code of Criminal Procedure (CrPC), and Indian Evidence Act. These laws, effective from July 1, 2024, are the Bharatiya Nyaya Sanhita, Bharatiya Nagarik…